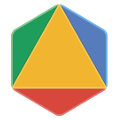 |
OR-Tools
8.1
|
Go to the documentation of this file.
19 #include "absl/container/flat_hash_set.h"
20 #include "absl/strings/str_cat.h"
21 #include "absl/strings/str_format.h"
22 #include "absl/strings/str_join.h"
62 result.
values.push_back(included_min);
63 result.
values.push_back(included_max);
72 result.
values.push_back(0);
73 result.
values.push_back(1);
118 if (!domain.
values.empty()) {
144 if (interval_min > interval_max) {
150 values.push_back(interval_min);
151 values.push_back(interval_max);
154 if (
values[0] >= interval_min &&
values[1] <= interval_max)
return false;
169 std::vector<int64> new_values;
170 new_values.reserve(
values.size());
171 bool changed =
false;
173 if (val > interval_max) {
177 if (val >= interval_min &&
178 (new_values.empty() || val != new_values.back())) {
179 new_values.push_back(val);
196 for (
const int64 v : integers) {
197 if (v >= dmin && v <= dmax)
values.push_back(v);
218 absl::flat_hash_set<int64> other_values(integers.begin(), integers.end());
219 std::vector<int64> new_values;
221 bool changed =
false;
224 if (new_values.empty() || val != new_values.back()) {
225 new_values.push_back(val);
278 bool IntervalOverlapValues(
int64 lb,
int64 ub,
279 const std::vector<int64>& values) {
295 return IntervalOverlapValues(
values[0],
values[1], vec);
298 const std::vector<int64>& to_scan =
300 const absl::flat_hash_set<int64> container =
301 values.size() <= vec.size()
302 ? absl::flat_hash_set<int64>(vec.begin(), vec.end())
303 : absl::flat_hash_set<int64>(
values.begin(),
values.end());
321 return !(dub < lb || dlb > ub);
323 return IntervalOverlapValues(lb, ub,
values);
329 if (other.
values.empty()) {
375 return absl::StrFormat(
"[%d..%d]",
values[0],
values[1]);
377 }
else if (
values.size() == 1) {
378 return absl::StrCat(
values.back());
380 return absl::StrFormat(
"[%s]", absl::StrJoin(
values,
", "));
396 result.
values.push_back(imin);
397 result.
values.push_back(imax);
437 if (domain.
values.empty()) {
450 return absl::StrFormat(
"% d",
values[0]);
452 return absl::StrFormat(
"[%d..%d]",
values[0],
values[1]);
454 return absl::StrFormat(
"[%s]", absl::StrJoin(
values,
", "));
460 std::string result =
"[";
461 for (
int i = 0; i <
variables.size(); ++i) {
463 result.append(i !=
variables.size() - 1 ?
", " :
"]");
468 return "VoidArgument";
470 LOG(
FATAL) <<
"Unhandled case in DebugString " <<
static_cast<int>(
type);
510 if (!domain.HasOneValue()) {
520 if (!
var->domain.HasOneValue()) {
584 IntegerVariable::IntegerVariable(
const std::string& name_,
585 const Domain& domain_,
bool temporary_)
586 :
name(name_), domain(domain_), temporary(temporary_), active(true) {
587 if (!domain.is_interval) {
593 const Domain& other_domain,
bool other_temporary) {
608 active ?
"" :
" [removed during presolve]");
616 const std::string presolve_status_str =
619 :
"[removed during presolve]");
621 strong, presolve_status_str);
634 type =
"false_constraint";
667 std::vector<Annotation> args) {
729 std::vector<IntegerVariable*>*
const vars)
const {
731 ann.AppendAllIntegerVariables(vars);
759 std::string result =
"[";
760 for (
int i = 0; i <
variables.size(); ++i) {
762 result.append(i !=
variables.size() - 1 ?
", " :
"]");
770 LOG(
FATAL) <<
"Unhandled case in DebugString " <<
static_cast<int>(
type);
791 const std::string&
name, std::vector<Bounds>
bounds,
811 return absl::StrFormat(
"output_var(%s)",
variable->
name);
813 return absl::StrFormat(
"output_array([%s] [%s])",
827 const Domain& domain,
bool defined) {
829 variables_.push_back(
var);
837 variables_.push_back(
var);
842 std::vector<Argument> arguments,
bool is_domain) {
844 new Constraint(
id, std::move(arguments), is_domain);
845 constraints_.push_back(constraint);
849 std::vector<Argument> arguments) {
850 AddConstraint(
id, std::move(arguments),
false);
854 output_.push_back(std::move(output));
859 search_annotations_ = std::move(search_annotations);
863 std::vector<Annotation> search_annotations) {
866 search_annotations_ = std::move(search_annotations);
870 std::vector<Annotation> search_annotations) {
873 search_annotations_ = std::move(search_annotations);
877 std::string output = absl::StrFormat(
"Model %s\nVariables\n", name_);
878 for (
int i = 0; i < variables_.size(); ++i) {
879 absl::StrAppendFormat(&output,
" %s\n", variables_[i]->
DebugString());
881 output.append(
"Constraints\n");
882 for (
int i = 0; i < constraints_.size(); ++i) {
883 if (constraints_[i] !=
nullptr) {
884 absl::StrAppendFormat(&output,
" %s\n", constraints_[i]->
DebugString());
888 absl::StrAppendFormat(&output,
"%s %s\n %s\n",
892 absl::StrAppendFormat(&output,
"Satisfy\n %s\n",
895 output.append(
"Output\n");
896 for (
int i = 0; i < output_.size(); ++i) {
897 absl::StrAppendFormat(&output,
" %s\n", output_[i].
DebugString());
905 if (
var->domain.empty()) {
910 if (
ct->type ==
"false_constraint") {
922 for (
const auto& it : constraints_per_type_) {
923 FZLOG <<
" - " << it.first <<
": " << it.second.size() <<
FZENDL;
925 if (model_.objective() ==
nullptr) {
928 FZLOG <<
" - " << (model_.maximize() ?
"Maximization" :
"Minimization")
934 constraints_per_type_.clear();
935 constraints_per_variables_.clear();
937 if (
ct !=
nullptr &&
ct->active) {
938 constraints_per_type_[
ct->type].push_back(
ct);
939 absl::flat_hash_set<const IntegerVariable*> marked;
946 constraints_per_variables_[
var].push_back(
ct);
std::vector< Bounds > bounds
static Argument Interval(int64 imin, int64 imax)
bool OverlapsIntList(const std::vector< int64 > &vec) const
static Domain IntegerList(std::vector< int64 > values)
static Domain SetOfAllInt64()
static Annotation FunctionCallWithArguments(const std::string &id, std::vector< Annotation > args)
#define CHECK_GE(val1, val2)
void Minimize(IntegerVariable *obj, std::vector< Annotation > search_annotations)
static Argument IntVarRefArray(std::vector< IntegerVariable * > vars)
std::vector< Annotation > annotations
std::string DebugString() const
bool IntersectWithSingleton(int64 value)
#define CHECK_LT(val1, val2)
static Annotation String(const std::string &str)
The vehicle routing library lets one model and solve generic vehicle routing problems ranging from th...
void Satisfy(std::vector< Annotation > search_annotations)
static const int64 kint64min
static Annotation Variable(IntegerVariable *const var)
void AddConstraint(const std::string &id, std::vector< Argument > arguments, bool is_domain)
bool IntersectWithDomain(const Domain &domain)
std::vector< Domain > domains
static Domain SetOfBoolean()
std::vector< int64 > values
static Domain SetOfIntegerValue(int64 value)
static Annotation VariableList(std::vector< IntegerVariable * > variables)
std::string DebugString() const
std::vector< IntegerVariable * > variables
static Domain IntegerValue(int64 value)
bool IsFunctionCallWithIdentifier(const std::string &identifier) const
std::vector< int64 > values
std::string DebugString() const
static Annotation FunctionCall(const std::string &id)
static Annotation IntegerValue(int64 value)
IntegerVariable * Var() const
static Domain EmptyDomain()
int64 ValueAt(int pos) const
bool IsInconsistent() const
std::string JoinNameFieldPtr(const std::vector< T > &v, const std::string &separator)
void STLSortAndRemoveDuplicates(T *v, const LessFunc &less_func)
static Argument VoidArgument()
static Argument IntegerList(std::vector< int64 > values)
std::vector< IntegerVariable * > flat_variables
std::string DebugString() const
bool presolve_propagation_done
#define DCHECK(condition)
std::string DebugString() const
static Domain SetOfInterval(int64 included_min, int64 included_max)
bool Contains(int64 value) const
std::string DebugString() const
static SolutionOutputSpecs VoidOutput()
bool OverlapsIntInterval(int64 lb, int64 ub) const
IntegerVariable * VarAt(int pos) const
std::string DebugString() const
static Domain SetOfIntegerList(std::vector< int64 > values)
void AppendAllIntegerVariables(std::vector< IntegerVariable * > *vars) const
static Argument DomainList(std::vector< Domain > domains)
static Argument IntVarRef(IntegerVariable *const var)
static SolutionOutputSpecs SingleVariable(const std::string &name, IntegerVariable *variable, bool display_as_boolean)
bool OverlapsDomain(const Domain &other) const
static SolutionOutputSpecs MultiDimensionalArray(const std::string &name, std::vector< Bounds > bounds, std::vector< IntegerVariable * > flat_variables, bool display_as_boolean)
std::string JoinDebugString(const std::vector< T > &v, const std::string &separator)
IntegerVariable * AddVariable(const std::string &name, const Domain &domain, bool defined)
void PrintStatistics() const
static Domain Interval(int64 included_min, int64 included_max)
IntegerVariable * variable
std::string DebugString() const
bool Merge(const std::string &other_name, const Domain &other_domain, bool other_temporary)
static Annotation Identifier(const std::string &id)
bool IsArrayOfValues() const
static Annotation Empty()
void FlattenAnnotations(const Annotation &ann, std::vector< Annotation > *out)
void RemoveArg(int arg_pos)
void STLDeleteElements(T *container)
void AddOutput(SolutionOutputSpecs output)
static Annotation AnnotationList(std::vector< Annotation > list)
static Argument IntegerValue(int64 value)
std::vector< Argument > arguments
bool IntersectWithInterval(int64 interval_min, int64 interval_max)
bool Contains(int64 value) const
std::vector< IntegerVariable * > variables
static Argument FromDomain(const Domain &domain)
bool RemoveValue(int64 value)
static Annotation Interval(int64 interval_min, int64 interval_max)
void Maximize(IntegerVariable *obj, std::vector< Annotation > search_annotations)
bool IntersectWithListOfIntegers(const std::vector< int64 > &integers)
static const int64 kint64max
IntegerVariable * AddConstant(int64 value)
bool ContainsKey(const Collection &collection, const Key &key)