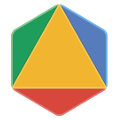 |
OR-Tools
8.1
|
Go to the documentation of this file.
14 #ifndef OR_TOOLS_BASE_LOGGING_H_
15 #define OR_TOOLS_BASE_LOGGING_H_
26 #if defined(__GNUC__) && defined(__linux__)
39 #define ABSL_DIE_IF_NULL CHECK_NOTNULL
40 #define CHECK_OK(x) CHECK((x).ok())
46 #define GLOG_MSVC_PUSH_DISABLE_WARNING(n) \
47 __pragma(warning(push)) __pragma(warning(disable : n))
48 #define GLOG_MSVC_POP_WARNING() __pragma(warning(pop))
49 #define ATTRIBUTE_NOINLINE
50 #define ATTRIBUTE_NORETURN __declspec(noreturn)
52 #define GLOG_MSVC_PUSH_DISABLE_WARNING(n)
53 #define GLOG_MSVC_POP_WARNING()
54 #define ATTRIBUTE_NOINLINE __attribute__((noinline))
55 #define ATTRIBUTE_NORETURN __attribute__((noreturn))
67 #ifndef GOOGLE_STRIP_LOG
68 #define GOOGLE_STRIP_LOG 0
76 #ifndef GOOGLE_PREDICT_BRANCH_NOT_TAKEN
77 #if !defined(_MSC_VER)
78 #define GOOGLE_PREDICT_BRANCH_NOT_TAKEN(x) (__builtin_expect(x, 0))
80 #define GOOGLE_PREDICT_BRANCH_NOT_TAKEN(x) x
84 #ifndef GOOGLE_PREDICT_FALSE
85 #if !defined(_MSC_VER)
86 #define GOOGLE_PREDICT_FALSE(x) (__builtin_expect(x, 0))
88 #define GOOGLE_PREDICT_FALSE(x) x
92 #ifndef GOOGLE_PREDICT_TRUE
93 #if !defined(_MSC_VER)
94 #define GOOGLE_PREDICT_TRUE(x) (__builtin_expect(!!(x), 1))
96 #define GOOGLE_PREDICT_TRUE(x) x
306 #if GOOGLE_STRIP_LOG == 0
307 #define COMPACT_GOOGLE_LOG_INFO google::LogMessage(__FILE__, __LINE__)
308 #define LOG_TO_STRING_INFO(message) \
309 google::LogMessage(__FILE__, __LINE__, google::GLOG_INFO, message)
311 #define COMPACT_GOOGLE_LOG_INFO google::NullStream()
312 #define LOG_TO_STRING_INFO(message) google::NullStream()
315 #if GOOGLE_STRIP_LOG <= 1
316 #define COMPACT_GOOGLE_LOG_WARNING \
317 google::LogMessage(__FILE__, __LINE__, google::GLOG_WARNING)
318 #define LOG_TO_STRING_WARNING(message) \
319 google::LogMessage(__FILE__, __LINE__, google::GLOG_WARNING, message)
321 #define COMPACT_GOOGLE_LOG_WARNING google::NullStream()
322 #define LOG_TO_STRING_WARNING(message) google::NullStream()
325 #if GOOGLE_STRIP_LOG <= 2
326 #define COMPACT_GOOGLE_LOG_ERROR \
327 google::LogMessage(__FILE__, __LINE__, google::GLOG_ERROR)
328 #define LOG_TO_STRING_ERROR(message) \
329 google::LogMessage(__FILE__, __LINE__, google::GLOG_ERROR, message)
331 #define COMPACT_GOOGLE_LOG_ERROR google::NullStream()
332 #define LOG_TO_STRING_ERROR(message) google::NullStream()
335 #if GOOGLE_STRIP_LOG <= 3
336 #define COMPACT_GOOGLE_LOG_FATAL google::LogMessageFatal(__FILE__, __LINE__)
337 #define LOG_TO_STRING_FATAL(message) \
338 google::LogMessage(__FILE__, __LINE__, google::GLOG_FATAL, message)
340 #define COMPACT_GOOGLE_LOG_FATAL google::NullStreamFatal()
341 #define LOG_TO_STRING_FATAL(message) google::NullStreamFatal()
344 #if defined(NDEBUG) && !defined(DCHECK_ALWAYS_ON)
345 #define DCHECK_IS_ON() 0
347 #define DCHECK_IS_ON() 1
353 #define COMPACT_GOOGLE_LOG_DFATAL COMPACT_GOOGLE_LOG_ERROR
354 #elif GOOGLE_STRIP_LOG <= 3
355 #define COMPACT_GOOGLE_LOG_DFATAL \
356 google::LogMessage(__FILE__, __LINE__, google::GLOG_FATAL)
358 #define COMPACT_GOOGLE_LOG_DFATAL google::NullStreamFatal()
361 #define GOOGLE_LOG_INFO(counter) \
362 google::LogMessage(__FILE__, __LINE__, google::GLOG_INFO, counter, \
363 &google::LogMessage::SendToLog)
364 #define SYSLOG_INFO(counter) \
365 google::LogMessage(__FILE__, __LINE__, google::GLOG_INFO, counter, \
366 &google::LogMessage::SendToSyslogAndLog)
367 #define GOOGLE_LOG_WARNING(counter) \
368 google::LogMessage(__FILE__, __LINE__, google::GLOG_WARNING, counter, \
369 &google::LogMessage::SendToLog)
370 #define SYSLOG_WARNING(counter) \
371 google::LogMessage(__FILE__, __LINE__, google::GLOG_WARNING, counter, \
372 &google::LogMessage::SendToSyslogAndLog)
373 #define GOOGLE_LOG_ERROR(counter) \
374 google::LogMessage(__FILE__, __LINE__, google::GLOG_ERROR, counter, \
375 &google::LogMessage::SendToLog)
376 #define SYSLOG_ERROR(counter) \
377 google::LogMessage(__FILE__, __LINE__, google::GLOG_ERROR, counter, \
378 &google::LogMessage::SendToSyslogAndLog)
379 #define GOOGLE_LOG_FATAL(counter) \
380 google::LogMessage(__FILE__, __LINE__, google::GLOG_FATAL, counter, \
381 &google::LogMessage::SendToLog)
382 #define SYSLOG_FATAL(counter) \
383 google::LogMessage(__FILE__, __LINE__, google::GLOG_FATAL, counter, \
384 &google::LogMessage::SendToSyslogAndLog)
385 #define GOOGLE_LOG_DFATAL(counter) \
386 google::LogMessage(__FILE__, __LINE__, google::DFATAL_LEVEL, counter, \
387 &google::LogMessage::SendToLog)
388 #define SYSLOG_DFATAL(counter) \
389 google::LogMessage(__FILE__, __LINE__, google::DFATAL_LEVEL, counter, \
390 &google::LogMessage::SendToSyslogAndLog)
392 #if defined(WIN32) || defined(_WIN32) || defined(__WIN32__) || \
393 defined(__CYGWIN__) || defined(__CYGWIN32__)
395 #define LOG_SYSRESULT(result) \
396 if (FAILED(HRESULT_FROM_WIN32(result))) { \
397 LPSTR message = NULL; \
398 LPSTR msg = reinterpret_cast<LPSTR>(&message); \
399 DWORD message_length = FormatMessageA( \
400 FORMAT_MESSAGE_ALLOCATE_BUFFER | FORMAT_MESSAGE_FROM_SYSTEM, 0, \
401 result, 0, msg, 100, NULL); \
402 if (message_length > 0) { \
403 google::LogMessage(__FILE__, __LINE__, google::GLOG_ERROR, 0, \
404 &google::LogMessage::SendToLog) \
406 << reinterpret_cast<const char*>(message); \
407 LocalFree(message); \
420 #define LOG(severity) COMPACT_GOOGLE_LOG_##severity.stream()
421 #define SYSLOG(severity) SYSLOG_##severity(0).stream()
445 #define LOG_TO_SINK(sink, severity) \
446 google::LogMessage(__FILE__, __LINE__, google::GLOG_##severity, \
447 static_cast<google::LogSink*>(sink), true) \
449 #define LOG_TO_SINK_BUT_NOT_TO_LOGFILE(sink, severity) \
450 google::LogMessage(__FILE__, __LINE__, google::GLOG_##severity, \
451 static_cast<google::LogSink*>(sink), false) \
464 #define LOG_TO_STRING(severity, message) \
465 LOG_TO_STRING_##severity(static_cast<string*>(message)).stream()
475 #define LOG_STRING(severity, outvec) \
476 LOG_TO_STRING_##severity(static_cast<std::vector<std::string>*>(outvec)) \
479 #define LOG_IF(severity, condition) \
480 static_cast<void>(0), \
481 !(condition) ? (void)0 : google::LogMessageVoidify() & LOG(severity)
482 #define SYSLOG_IF(severity, condition) \
483 static_cast<void>(0), \
484 !(condition) ? (void)0 : google::LogMessageVoidify() & SYSLOG(severity)
486 #define LOG_ASSERT(condition) \
487 LOG_IF(FATAL, !(condition)) << "Assert failed: " #condition
488 #define SYSLOG_ASSERT(condition) \
489 SYSLOG_IF(FATAL, !(condition)) << "Assert failed: " #condition
495 #define CHECK(condition) \
496 LOG_IF(FATAL, GOOGLE_PREDICT_BRANCH_NOT_TAKEN(!(condition))) \
497 << "Check failed: " #condition " "
505 operator bool()
const {
544 template <
typename T>
556 const signed char& v);
559 const unsigned char& v);
562 template <
typename T1,
typename T2>
591 std::ostream* ForVar2();
593 std::string* NewString();
596 std::ostringstream* stream_;
601 template <
typename T1,
typename T2>
603 const char* exprtext) {
614 #define DEFINE_CHECK_OP_IMPL(name, op) \
615 template <typename T1, typename T2> \
616 inline std::string* name##Impl(const T1& v1, const T2& v2, \
617 const char* exprtext) { \
618 if (GOOGLE_PREDICT_TRUE(v1 op v2)) \
621 return MakeCheckOpString(v1, v2, exprtext); \
623 inline std::string* name##Impl(int v1, int v2, const char* exprtext) { \
624 return name##Impl<int, int>(v1, v2, exprtext); \
637 #undef DEFINE_CHECK_OP_IMPL
642 #if defined(STATIC_ANALYSIS)
644 #define CHECK_OP_LOG(name, op, val1, val2, log) CHECK((val1)op(val2))
655 typedef std::string _Check_string;
656 #define CHECK_OP_LOG(name, op, val1, val2, log) \
657 while (google::_Check_string* _result = google::Check##name##Impl( \
658 google::GetReferenceableValue(val1), \
659 google::GetReferenceableValue(val2), #val1 " " #op " " #val2)) \
660 log(__FILE__, __LINE__, google::CheckOpString(_result)).stream()
664 #define CHECK_OP_LOG(name, op, val1, val2, log) \
665 while (google::CheckOpString _result = google::Check##name##Impl( \
666 google::GetReferenceableValue(val1), \
667 google::GetReferenceableValue(val2), #val1 " " #op " " #val2)) \
668 log(__FILE__, __LINE__, _result).stream()
669 #endif // STATIC_ANALYSIS, DCHECK_IS_ON()
671 #if GOOGLE_STRIP_LOG <= 3
672 #define CHECK_OP(name, op, val1, val2) \
673 CHECK_OP_LOG(name, op, val1, val2, google::LogMessageFatal)
675 #define CHECK_OP(name, op, val1, val2) \
676 CHECK_OP_LOG(name, op, val1, val2, google::NullStreamFatal)
677 #endif // STRIP_LOG <= 3
697 #define CHECK_EQ(val1, val2) CHECK_OP(_EQ, ==, val1, val2)
698 #define CHECK_NE(val1, val2) CHECK_OP(_NE, !=, val1, val2)
699 #define CHECK_LE(val1, val2) CHECK_OP(_LE, <=, val1, val2)
700 #define CHECK_LT(val1, val2) CHECK_OP(_LT, <, val1, val2)
701 #define CHECK_GE(val1, val2) CHECK_OP(_GE, >=, val1, val2)
702 #define CHECK_GT(val1, val2) CHECK_OP(_GT, >, val1, val2)
707 #define CHECK_NOTNULL(val) \
708 google::CheckNotNull(__FILE__, __LINE__, "'" #val "' Must be non NULL", (val))
712 #define DECLARE_CHECK_STROP_IMPL(func, expected) \
713 GOOGLE_GLOG_DLL_DECL std::string* Check##func##expected##Impl( \
714 const char* s1, const char* s2, const char* names);
719 #undef DECLARE_CHECK_STROP_IMPL
723 #define CHECK_STROP(func, op, expected, s1, s2) \
724 while (google::CheckOpString _result = google::Check##func##expected##Impl( \
725 (s1), (s2), #s1 " " #op " " #s2)) \
726 LOG(FATAL) << *_result.str_
735 #define CHECK_STREQ(s1, s2) CHECK_STROP(strcmp, ==, true, s1, s2)
736 #define CHECK_STRNE(s1, s2) CHECK_STROP(strcmp, !=, false, s1, s2)
737 #define CHECK_STRCASEEQ(s1, s2) CHECK_STROP(strcasecmp, ==, true, s1, s2)
738 #define CHECK_STRCASENE(s1, s2) CHECK_STROP(strcasecmp, !=, false, s1, s2)
740 #define CHECK_INDEX(I, A) CHECK(I < (sizeof(A) / sizeof(A[0])))
741 #define CHECK_BOUND(B, A) CHECK(B <= (sizeof(A) / sizeof(A[0])))
743 #define CHECK_DOUBLE_EQ(val1, val2) \
745 CHECK_LE((val1), (val2) + 0.000000000000001L); \
746 CHECK_GE((val1), (val2)-0.000000000000001L); \
749 #define CHECK_NEAR(val1, val2, margin) \
751 CHECK_LE((val1), (val2) + (margin)); \
752 CHECK_GE((val1), (val2) - (margin)); \
761 #define PLOG(severity) GOOGLE_PLOG(severity, 0).stream()
763 #define GOOGLE_PLOG(severity, counter) \
764 google::ErrnoLogMessage(__FILE__, __LINE__, google::GLOG_##severity, \
765 counter, &google::LogMessage::SendToLog)
767 #define PLOG_IF(severity, condition) \
768 static_cast<void>(0), \
769 !(condition) ? (void)0 : google::LogMessageVoidify() & PLOG(severity)
774 #define PCHECK(condition) \
775 PLOG_IF(FATAL, GOOGLE_PREDICT_BRANCH_NOT_TAKEN(!(condition))) \
776 << "Check failed: " #condition " "
786 #define CHECK_ERR(invocation) \
787 PLOG_IF(FATAL, GOOGLE_PREDICT_BRANCH_NOT_TAKEN((invocation) == -1)) \
792 #define LOG_EVERY_N_VARNAME(base, line) LOG_EVERY_N_VARNAME_CONCAT(base, line)
793 #define LOG_EVERY_N_VARNAME_CONCAT(base, line) base##line
795 #define LOG_OCCURRENCES LOG_EVERY_N_VARNAME(occurrences_, __LINE__)
796 #define LOG_OCCURRENCES_MOD_N LOG_EVERY_N_VARNAME(occurrences_mod_n_, __LINE__)
798 #define SOME_KIND_OF_LOG_EVERY_N(severity, n, what_to_do) \
799 static int LOG_OCCURRENCES = 0, LOG_OCCURRENCES_MOD_N = 0; \
801 if (++LOG_OCCURRENCES_MOD_N > n) LOG_OCCURRENCES_MOD_N -= n; \
802 if (LOG_OCCURRENCES_MOD_N == 1) \
803 google::LogMessage(__FILE__, __LINE__, google::GLOG_##severity, \
804 LOG_OCCURRENCES, &what_to_do) \
807 #define SOME_KIND_OF_LOG_IF_EVERY_N(severity, condition, n, what_to_do) \
808 static int LOG_OCCURRENCES = 0, LOG_OCCURRENCES_MOD_N = 0; \
811 ((LOG_OCCURRENCES_MOD_N = (LOG_OCCURRENCES_MOD_N + 1) % n) == (1 % n))) \
812 google::LogMessage(__FILE__, __LINE__, google::GLOG_##severity, \
813 LOG_OCCURRENCES, &what_to_do) \
816 #define SOME_KIND_OF_PLOG_EVERY_N(severity, n, what_to_do) \
817 static int LOG_OCCURRENCES = 0, LOG_OCCURRENCES_MOD_N = 0; \
819 if (++LOG_OCCURRENCES_MOD_N > n) LOG_OCCURRENCES_MOD_N -= n; \
820 if (LOG_OCCURRENCES_MOD_N == 1) \
821 google::ErrnoLogMessage(__FILE__, __LINE__, google::GLOG_##severity, \
822 LOG_OCCURRENCES, &what_to_do) \
825 #define SOME_KIND_OF_LOG_FIRST_N(severity, n, what_to_do) \
826 static int LOG_OCCURRENCES = 0; \
827 if (LOG_OCCURRENCES <= n) ++LOG_OCCURRENCES; \
828 if (LOG_OCCURRENCES <= n) \
829 google::LogMessage(__FILE__, __LINE__, google::GLOG_##severity, \
830 LOG_OCCURRENCES, &what_to_do) \
833 namespace logging_internal {
839 #define LOG_EVERY_N(severity, n) \
840 SOME_KIND_OF_LOG_EVERY_N(severity, (n), google::LogMessage::SendToLog)
842 #define SYSLOG_EVERY_N(severity, n) \
843 SOME_KIND_OF_LOG_EVERY_N(severity, (n), \
844 google::LogMessage::SendToSyslogAndLog)
846 #define PLOG_EVERY_N(severity, n) \
847 SOME_KIND_OF_PLOG_EVERY_N(severity, (n), google::LogMessage::SendToLog)
849 #define LOG_FIRST_N(severity, n) \
850 SOME_KIND_OF_LOG_FIRST_N(severity, (n), google::LogMessage::SendToLog)
852 #define LOG_IF_EVERY_N(severity, condition, n) \
853 SOME_KIND_OF_LOG_IF_EVERY_N(severity, (condition), (n), \
854 google::LogMessage::SendToLog)
864 #define COMPACT_GOOGLE_LOG_0 COMPACT_GOOGLE_LOG_ERROR
865 #define SYSLOG_0 SYSLOG_ERROR
866 #define LOG_TO_STRING_0 LOG_TO_STRING_ERROR
875 #define DLOG(severity) LOG(severity)
876 #define DVLOG(verboselevel) VLOG(verboselevel)
877 #define DLOG_IF(severity, condition) LOG_IF(severity, condition)
878 #define DLOG_EVERY_N(severity, n) LOG_EVERY_N(severity, n)
879 #define DLOG_IF_EVERY_N(severity, condition, n) \
880 LOG_IF_EVERY_N(severity, condition, n)
881 #define DLOG_ASSERT(condition) LOG_ASSERT(condition)
884 #define DCHECK(condition) CHECK(condition)
885 #define DCHECK_EQ(val1, val2) CHECK_EQ(val1, val2)
886 #define DCHECK_NE(val1, val2) CHECK_NE(val1, val2)
887 #define DCHECK_LE(val1, val2) CHECK_LE(val1, val2)
888 #define DCHECK_LT(val1, val2) CHECK_LT(val1, val2)
889 #define DCHECK_GE(val1, val2) CHECK_GE(val1, val2)
890 #define DCHECK_GT(val1, val2) CHECK_GT(val1, val2)
891 #define DCHECK_NOTNULL(val) CHECK_NOTNULL(val)
892 #define DCHECK_STREQ(str1, str2) CHECK_STREQ(str1, str2)
893 #define DCHECK_STRCASEEQ(str1, str2) CHECK_STRCASEEQ(str1, str2)
894 #define DCHECK_STRNE(str1, str2) CHECK_STRNE(str1, str2)
895 #define DCHECK_STRCASENE(str1, str2) CHECK_STRCASENE(str1, str2)
897 #else // !DCHECK_IS_ON()
899 #define DLOG(severity) \
900 static_cast<void>(0), \
901 true ? (void)0 : google::LogMessageVoidify() & LOG(severity)
903 #define DVLOG(verboselevel) \
904 static_cast<void>(0), (true || !VLOG_IS_ON(verboselevel)) \
906 : google::LogMessageVoidify() & LOG(INFO)
908 #define DLOG_IF(severity, condition) \
909 static_cast<void>(0), (true || !(condition)) \
911 : google::LogMessageVoidify() & LOG(severity)
913 #define DLOG_EVERY_N(severity, n) \
914 static_cast<void>(0), \
915 true ? (void)0 : google::LogMessageVoidify() & LOG(severity)
917 #define DLOG_IF_EVERY_N(severity, condition, n) \
918 static_cast<void>(0), (true || !(condition)) \
920 : google::LogMessageVoidify() & LOG(severity)
922 #define DLOG_ASSERT(condition) \
923 static_cast<void>(0), true ? (void)0 : LOG_ASSERT(condition)
926 #define DCHECK(condition) \
927 GLOG_MSVC_PUSH_DISABLE_WARNING(4127) \
928 while (false) GLOG_MSVC_POP_WARNING() CHECK(condition)
930 #define DCHECK_EQ(val1, val2) \
931 GLOG_MSVC_PUSH_DISABLE_WARNING(4127) \
932 while (false) GLOG_MSVC_POP_WARNING() CHECK_EQ(val1, val2)
934 #define DCHECK_NE(val1, val2) \
935 GLOG_MSVC_PUSH_DISABLE_WARNING(4127) \
936 while (false) GLOG_MSVC_POP_WARNING() CHECK_NE(val1, val2)
938 #define DCHECK_LE(val1, val2) \
939 GLOG_MSVC_PUSH_DISABLE_WARNING(4127) \
940 while (false) GLOG_MSVC_POP_WARNING() CHECK_LE(val1, val2)
942 #define DCHECK_LT(val1, val2) \
943 GLOG_MSVC_PUSH_DISABLE_WARNING(4127) \
944 while (false) GLOG_MSVC_POP_WARNING() CHECK_LT(val1, val2)
946 #define DCHECK_GE(val1, val2) \
947 GLOG_MSVC_PUSH_DISABLE_WARNING(4127) \
948 while (false) GLOG_MSVC_POP_WARNING() CHECK_GE(val1, val2)
950 #define DCHECK_GT(val1, val2) \
951 GLOG_MSVC_PUSH_DISABLE_WARNING(4127) \
952 while (false) GLOG_MSVC_POP_WARNING() CHECK_GT(val1, val2)
956 #define DCHECK_NOTNULL(val) (val)
958 #define DCHECK_STREQ(str1, str2) \
959 GLOG_MSVC_PUSH_DISABLE_WARNING(4127) \
960 while (false) GLOG_MSVC_POP_WARNING() CHECK_STREQ(str1, str2)
962 #define DCHECK_STRCASEEQ(str1, str2) \
963 GLOG_MSVC_PUSH_DISABLE_WARNING(4127) \
964 while (false) GLOG_MSVC_POP_WARNING() CHECK_STRCASEEQ(str1, str2)
966 #define DCHECK_STRNE(str1, str2) \
967 GLOG_MSVC_PUSH_DISABLE_WARNING(4127) \
968 while (false) GLOG_MSVC_POP_WARNING() CHECK_STRNE(str1, str2)
970 #define DCHECK_STRCASENE(str1, str2) \
971 GLOG_MSVC_PUSH_DISABLE_WARNING(4127) \
972 while (false) GLOG_MSVC_POP_WARNING() CHECK_STRCASENE(str1, str2)
974 #endif // DCHECK_IS_ON()
978 #define VLOG(verboselevel) LOG_IF(INFO, VLOG_IS_ON(verboselevel))
980 #define VLOG_IF(verboselevel, condition) \
981 LOG_IF(INFO, (condition) && VLOG_IS_ON(verboselevel))
983 #define VLOG_EVERY_N(verboselevel, n) \
984 LOG_IF_EVERY_N(INFO, VLOG_IS_ON(verboselevel), n)
986 #define VLOG_IF_EVERY_N(verboselevel, condition, n) \
987 LOG_IF_EVERY_N(INFO, (condition) && VLOG_IS_ON(verboselevel), n)
989 namespace base_logging {
1003 size_t pcount()
const {
return pptr() - pbase(); }
1004 char*
pbase()
const {
return std::streambuf::pbase(); }
1040 : std::ostream(NULL), streambuf_(buf, len), ctr_(ctr), self_(this) {
1044 int ctr()
const {
return ctr_; }
1049 size_t pcount()
const {
return streambuf_.pcount(); }
1050 char*
pbase()
const {
return streambuf_.pbase(); }
1051 char*
str()
const {
return pbase(); }
1066 SendMethod send_method);
1089 bool also_send_to_log);
1095 std::vector<std::string>* outvec);
1120 void SendToSyslogAndLog();
1125 std::ostream& stream();
1127 int preserved_errno()
const;
1130 static int64 num_messages(
int severity);
1136 void SendToSinkAndLog();
1140 void WriteToStringAndLog();
1142 void SaveOrSendToLog();
1176 inline void LogAtLevel(
int const severity, std::string
const& msg) {
1184 #define LOG_AT_LEVEL(severity) \
1185 google::LogMessage(__FILE__, __LINE__, severity).stream()
1196 #if (defined(__GXX_EXPERIMENTAL_CXX0X__) || __cplusplus >= 201103L || \
1197 (defined(_MSC_VER) && _MSC_VER >= 1900))
1206 template <
typename T>
1209 LogMessageFatal(
file, line,
new std::string(names));
1211 return std::forward<T>(t);
1217 template <
typename T>
1273 const char* base_filename);
1282 const char* symlink_basename);
1297 const char* base_filename,
int line,
1298 const struct ::tm* tm_time,
const char*
message,
1299 size_t message_len) = 0;
1314 virtual void WaitTillSent();
1319 const struct ::tm* tm_time,
const char*
message,
1320 size_t message_len);
1334 const char* filename_extension);
1359 std::vector<std::string>* list);
1395 virtual void Write(
bool force_flush, time_t timestamp,
const char*
message,
1396 int message_len) = 0;
1446 :
LogMessage::LogStream(message_buffer_, 1, 0) {}
1453 char message_buffer_[2];
1478 #endif // OR_TOOLS_BASE_LOGGING_H_
void SetStderrLogging(LogSeverity min_severity)
void SetLogSymlink(LogSeverity severity, const char *symlink_basename)
void SetLogFilenameExtension(const char *ext)
void FixFlagsAndEnvironmentForSwig()
void MakeCheckOpValueString(std::ostream *os, const char &v)
#define GOOGLE_GLOG_DLL_DECL
void AddLogSink(LogSink *destination)
GOOGLE_GLOG_DLL_DECL Logger * GetLogger(LogSeverity level)
void RemoveLogSink(LogSink *destination)
const char * GetLogSeverityName(LogSeverity severity)
void ShutdownGoogleLogging()
const absl::string_view ToString(MPSolver::OptimizationProblemType optimization_problem_type)
void FlushLogFiles(LogSeverity min_severity)
void GetExistingTempDirectories(vector< string > *list)
const T & GetReferenceableValue(const T &t)
NullStreamFatal(const char *file, int line, const CheckOpString &result)
virtual int_type overflow(int_type ch)
std::string * MakeCheckOpString(const T1 &v1, const T2 &v2, const char *exprtext) ATTRIBUTE_NOINLINE
void FlushLogFilesUnsafe(LogSeverity min_severity)
GOOGLE_GLOG_DLL_DECL void SetLogger(LogSeverity level, Logger *logger)
int posix_strerror_r(int err, char *buf, size_t len)
ostream & operator<<(ostream &os, const PRIVATE_Counter &)
void operator&(std::ostream &)
#define ATTRIBUTE_NORETURN
T * CheckNotNull(const char *file, int line, const char *names, T *t)
ATTRIBUTE_NORETURN ~NullStreamFatal()
std::ostream & operator<<(std::ostream &out, const google::DummyClassToDefineOperator &)
NullStream(const char *, int, const CheckOpString &)
#define ATTRIBUTE_NOINLINE
virtual void Write(bool force_flush, time_t timestamp, const char *message, int message_len)=0
#define GOOGLE_PREDICT_BRANCH_NOT_TAKEN(x)
DEFINE_CHECK_OP_IMPL(Check_EQ,==) DEFINE_CHECK_OP_IMPL(Check_NE
void TestOnly_ClearLoggingDirectoriesList()
void SetLogDestination(LogSeverity severity, const char *base_filename)
#define GLOG_MSVC_PUSH_DISABLE_WARNING(n)
LogStream(char *buf, int len, int ctr)
LogStreamBuf(char *buf, int len)
std::string * NewString()
void InstallFailureFunction(void(*fail_func)())
ABSL_DECLARE_FLAG(bool, logtostderr)
#define DECLARE_CHECK_STROP_IMPL(func, expected)
CheckOpString(std::string *str)
void ReprintFatalMessage()
virtual uint32 LogSize()=0
const vector< string > & GetLoggingDirectories()
LogSeverity NormalizeSeverity(LogSeverity s)
virtual void send(LogSeverity severity, const char *full_filename, const char *base_filename, int line, const struct ::tm *tm_time, const char *message, size_t message_len)=0
void LogAtLevel(int const severity, std::string const &msg)
void InitGoogleLogging(const char *argv0)
#define GLOG_MSVC_POP_WARNING()
static const size_t kMaxLogMessageLen