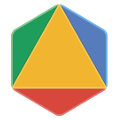 |
OR-Tools
8.1
|
Go to the documentation of this file.
14 #ifndef OR_TOOLS_FLATZINC_MODEL_H_
15 #define OR_TOOLS_FLATZINC_MODEL_H_
20 #include "absl/container/flat_hash_map.h"
118 bool Merge(
const std::string& other_name,
const Domain& other_domain,
119 bool other_temporary);
254 std::vector<Annotation> args);
299 const std::string&
name, std::vector<Bounds>
bounds,
318 : name_(
name), objective_(nullptr), maximize_(true) {}
329 void AddConstraint(
const std::string&
id, std::vector<Argument> arguments,
331 void AddConstraint(
const std::string&
id, std::vector<Argument> arguments);
347 const std::vector<IntegerVariable*>&
variables()
const {
return variables_; }
348 const std::vector<Constraint*>&
constraints()
const {
return constraints_; }
350 return search_annotations_;
357 const std::vector<SolutionOutputSpecs>&
output()
const {
return output_; }
370 const std::string&
name()
const {
return name_; }
373 const std::string name_;
376 std::vector<IntegerVariable*> variables_;
379 std::vector<Constraint*> constraints_;
384 std::vector<Annotation> search_annotations_;
385 std::vector<SolutionOutputSpecs> output_;
394 return constraints_per_variables_[
var].size();
401 std::map<std::string, std::vector<Constraint*>> constraints_per_type_;
402 absl::flat_hash_map<const IntegerVariable*, std::vector<Constraint*>>
403 constraints_per_variables_;
412 #endif // OR_TOOLS_FLATZINC_MODEL_H_
Bounds(int64 min_value_, int64 max_value_)
std::vector< Bounds > bounds
ModelStatistics(const Model &model)
static Argument Interval(int64 imin, int64 imax)
bool OverlapsIntList(const std::vector< int64 > &vec) const
static Domain IntegerList(std::vector< int64 > values)
static Domain SetOfAllInt64()
static Annotation FunctionCallWithArguments(const std::string &id, std::vector< Annotation > args)
Constraint(const std::string &t, std::vector< Argument > args, bool strong_propag)
void Minimize(IntegerVariable *obj, std::vector< Annotation > search_annotations)
IntegerVariable * objective() const
static Argument IntVarRefArray(std::vector< IntegerVariable * > vars)
std::vector< Annotation > annotations
std::string DebugString() const
const std::string & name() const
bool IntersectWithSingleton(int64 value)
static Annotation String(const std::string &str)
The vehicle routing library lets one model and solve generic vehicle routing problems ranging from th...
void Satisfy(std::vector< Annotation > search_annotations)
util::MutableVectorIteration< SolutionOutputSpecs > mutable_output()
static Annotation Variable(IntegerVariable *const var)
void AddConstraint(const std::string &id, std::vector< Argument > arguments, bool is_domain)
bool IntersectWithDomain(const Domain &domain)
std::vector< Domain > domains
static Domain SetOfBoolean()
std::vector< int64 > values
static Domain SetOfIntegerValue(int64 value)
static Annotation VariableList(std::vector< IntegerVariable * > variables)
std::string DebugString() const
std::vector< IntegerVariable * > variables
static Domain IntegerValue(int64 value)
bool IsFunctionCallWithIdentifier(const std::string &identifier) const
std::vector< int64 > values
std::string DebugString() const
static Annotation FunctionCall(const std::string &id)
const std::vector< IntegerVariable * > & variables() const
static Annotation IntegerValue(int64 value)
IntegerVariable * Var() const
static Domain EmptyDomain()
const std::vector< Annotation > & search_annotations() const
int64 ValueAt(int pos) const
bool IsInconsistent() const
static Argument VoidArgument()
Model(const std::string &name)
util::MutableVectorIteration< Annotation > mutable_search_annotations()
int NumVariableOccurrences(IntegerVariable *var)
static Argument IntegerList(std::vector< int64 > values)
std::vector< IntegerVariable * > flat_variables
std::string DebugString() const
bool presolve_propagation_done
std::string DebugString() const
static Domain SetOfInterval(int64 included_min, int64 included_max)
bool Contains(int64 value) const
std::string DebugString() const
static SolutionOutputSpecs VoidOutput()
bool OverlapsIntInterval(int64 lb, int64 ub) const
IntegerVariable * VarAt(int pos) const
std::string DebugString() const
static Domain SetOfIntegerList(std::vector< int64 > values)
void AppendAllIntegerVariables(std::vector< IntegerVariable * > *vars) const
void SetObjective(IntegerVariable *obj)
static Argument DomainList(std::vector< Domain > domains)
static Argument IntVarRef(IntegerVariable *const var)
static SolutionOutputSpecs SingleVariable(const std::string &name, IntegerVariable *variable, bool display_as_boolean)
bool OverlapsDomain(const Domain &other) const
static SolutionOutputSpecs MultiDimensionalArray(const std::string &name, std::vector< Bounds > bounds, std::vector< IntegerVariable * > flat_variables, bool display_as_boolean)
IntegerVariable * AddVariable(const std::string &name, const Domain &domain, bool defined)
void PrintStatistics() const
static Domain Interval(int64 included_min, int64 included_max)
IntegerVariable * variable
std::string DebugString() const
bool Merge(const std::string &other_name, const Domain &other_domain, bool other_temporary)
const std::vector< SolutionOutputSpecs > & output() const
static Annotation Identifier(const std::string &id)
bool IsArrayOfValues() const
static Annotation Empty()
void FlattenAnnotations(const Annotation &ann, std::vector< Annotation > *out)
void RemoveArg(int arg_pos)
void AddOutput(SolutionOutputSpecs output)
static Annotation AnnotationList(std::vector< Annotation > list)
static Argument IntegerValue(int64 value)
std::vector< Argument > arguments
bool IntersectWithInterval(int64 interval_min, int64 interval_max)
bool Contains(int64 value) const
std::vector< IntegerVariable * > variables
static Argument FromDomain(const Domain &domain)
bool RemoveValue(int64 value)
static Annotation Interval(int64 interval_min, int64 interval_max)
void Maximize(IntegerVariable *obj, std::vector< Annotation > search_annotations)
bool IntersectWithListOfIntegers(const std::vector< int64 > &integers)
const std::vector< Constraint * > & constraints() const
IntegerVariable * AddConstant(int64 value)