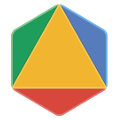 |
OR-Tools
8.1
|
Go to the documentation of this file.
17 #ifndef OR_TOOLS_SAT_CLAUSE_H_
18 #define OR_TOOLS_SAT_CLAUSE_H_
25 #include "absl/container/flat_hash_map.h"
26 #include "absl/container/flat_hash_set.h"
27 #include "absl/container/inlined_vector.h"
28 #include "absl/types/span.h"
60 void operator delete(
void* p) {
65 int size()
const {
return size_; }
66 int empty()
const {
return size_ == 0; }
70 const Literal*
const end()
const {
return &(literals_[size_]); }
86 return absl::Span<const Literal>(&(literals_[1]), size_ - 1);
90 absl::Span<const Literal>
AsSpan()
const {
91 return absl::Span<const Literal>(&(literals_[0]), size_);
117 Literal* literals() {
return &(literals_[0]); }
122 void Clear() { size_ = 0; }
126 void Rewrite(absl::Span<const Literal> new_clause) {
128 for (
const Literal l : new_clause) literals_[size_++] = l;
135 Literal literals_[0];
166 void Resize(
int num_variables);
170 absl::Span<const Literal>
Reason(
const Trail& trail,
171 int trail_index)
const final;
180 bool AddClause(absl::Span<const Literal> literals);
224 return &clauses_info_;
230 return num_inspected_clause_literals_;
240 drat_proof_handler_ = drat_proof_handler;
247 for (; to_minimize_index_ < clauses_.size(); ++to_minimize_index_) {
248 if (!clauses_[to_minimize_index_]->IsAttached())
continue;
250 return clauses_[to_minimize_index_++];
278 SatClause* clause, absl::Span<const Literal> new_clause);
313 return watchers_on_false_[false_literal.
Index()];
323 bool PropagateOnFalse(
Literal false_literal,
Trail* trail);
337 std::vector<SatClause*> reasons_;
342 bool is_clean_ =
true;
347 int64 num_inspected_clauses_;
348 int64 num_inspected_clause_literals_;
349 int64 num_watched_clauses_;
353 bool all_clauses_are_attached_ =
true;
362 std::vector<SatClause*> clauses_;
364 int to_minimize_index_ = 0;
367 absl::flat_hash_map<SatClause*, ClauseInfo> clauses_info_;
393 if (p.first > p.second) std::swap(p.first, p.second);
394 if (set_.find(p) == set_.end()) {
396 newly_added_.push_back(c);
403 const std::vector<BinaryClause>&
newly_added()
const {
return newly_added_; }
407 absl::flat_hash_set<std::pair<int, int>> set_;
408 std::vector<BinaryClause> newly_added_;
460 stats_(
"BinaryImplicationGraph"),
470 LOG(
INFO) <<
"num_redundant_implications " << num_redundant_implications_;
476 absl::Span<const Literal>
Reason(
const Trail& trail,
477 int trail_index)
const final;
480 void Resize(
int num_variables);
483 bool IsEmpty() {
return num_implications_ == 0 && at_most_ones_.
empty(); }
504 ABSL_MUST_USE_RESULT
bool AddAtMostOne(absl::Span<const Literal> at_most_one);
517 std::vector<Literal>* c);
521 const Trail& trail, std::vector<Literal>* c,
543 bool IsDag()
const {
return is_dag_; }
549 return reverse_topological_order_;
555 return implications_[l.
Index()];
562 if (l.
Index() >= representative_of_.
size())
return l;
589 int64 max_num_explored_nodes = 1e8);
601 const std::vector<Literal>& literals,
602 const std::vector<double>& lp_values);
622 CHECK_EQ(num_redundant_literals_ % 2, 0);
623 return num_redundant_literals_;
628 return num_redundant_implications_;
645 template <
typename Output>
650 absl::flat_hash_set<std::pair<LiteralIndex, LiteralIndex>>
652 for (LiteralIndex i(0); i < implications_.size(); ++i) {
654 for (
const Literal b : implications_[i]) {
660 duplicate_detection.insert({a.Index(), b.Index()}).second) {
661 out->AddBinaryClause(
a,
b);
668 drat_proof_handler_ = drat_proof_handler;
676 reasons_[trail_index] = new_reason.
Negated();
694 return estimated_sizes_[
literal.Index()];
707 BooleanVariable
var, std::deque<std::vector<Literal>>* postsolve_clauses);
716 bool FixLiteral(
Literal true_literal);
722 bool PropagateOnTrue(
Literal true_literal,
Trail* trail);
725 void RemoveRedundantLiterals(std::vector<Literal>* conflict);
729 void MarkDescendants(
Literal root);
735 std::vector<Literal> ExpandAtMostOne(
736 const absl::Span<const Literal> at_most_one);
739 std::vector<Literal> ExpandAtMostOneWithWeight(
740 const absl::Span<const Literal> at_most_one,
748 bool CleanUpAndAddAtMostOnes(
const int base_index);
758 std::deque<Literal> reasons_;
772 int64 num_implications_ = 0;
782 std::vector<Literal> at_most_one_buffer_;
785 std::vector<std::vector<Literal>> tmp_cuts_;
788 int64 num_propagations_ = 0;
789 int64 num_inspections_ = 0;
790 int64 num_minimization_ = 0;
791 int64 num_literals_removed_ = 0;
792 int64 num_redundant_implications_ = 0;
793 int64 num_redundant_literals_ = 0;
803 std::vector<Literal> dfs_stack_;
807 int64 work_done_in_mark_descendants_ = 0;
810 bool is_dag_ =
false;
811 std::vector<LiteralIndex> reverse_topological_order_;
816 std::vector<Literal> direct_implications_;
817 std::vector<Literal> direct_implications_of_negated_literal_;
823 int num_processed_fixed_variables_ = 0;
831 #endif // OR_TOOLS_SAT_CLAUSE_H_
const std::vector< LiteralIndex > & ReverseTopologicalOrder() const
bool IsRemovable(SatClause *const clause) const
absl::Span< const Literal > AsSpan() const
int64 literal_size() const
void MinimizeConflictFirstWithTransitiveReduction(const Trail &trail, std::vector< Literal > *c, SparseBitset< BooleanVariable > *marked, random_engine_t *random)
void Resize(int num_variables)
ABSL_MUST_USE_RESULT bool InprocessingFixLiteral(Literal true_literal)
#define IF_STATS_ENABLED(instructions)
std::string DebugString() const
absl::Span< const Literal > Reason(const Trail &trail, int trail_index) const final
const LiteralIndex kNoLiteralIndex(-1)
bool RemoveFixedLiteralsAndTestIfTrue(const VariablesAssignment &assignment)
int64 literal_size() const
bool FindFailedLiteralAroundVar(BooleanVariable var, bool *is_unsat)
absl::Span< const Literal > Reason(const Trail &trail, int trail_index) const final
bool DetectEquivalences(bool log_info=false)
absl::Span< const Literal > PropagationReason() const
int64 num_propagations() const
void InprocessingRemoveClause(SatClause *clause)
int64 num_redundant_implications() const
const std::vector< BinaryClause > & newly_added() const
bool Propagate(Trail *trail) final
bool Propagate(Trail *trail) final
const std::vector< std::vector< Literal > > & GenerateAtMostOnesWithLargeWeight(const std::vector< Literal > &literals, const std::vector< double > &lp_values)
The vehicle routing library lets one model and solve generic vehicle routing problems ranging from th...
bool LiteralIsTrue(Literal literal) const
bool ComputeTransitiveReduction(bool log_info=false)
int64 num_inspections() const
~BinaryImplicationGraph() override
void ChangeReason(int trail_index, Literal new_reason)
void ResetToMinimizeIndex()
void RemoveBooleanVariable(BooleanVariable var, std::deque< std::vector< Literal >> *postsolve_clauses)
void ExtractAllBinaryClauses(Output *out) const
A simple class to enforce both an elapsed time limit and a deterministic time limit in the same threa...
const std::vector< SatClause * > & AllClausesInCreationOrder() const
bool IsRedundant(Literal l) const
BinaryImplicationGraph(Model *model)
void DeleteRemovedClauses()
const std::vector< Literal > & DirectImplications(Literal literal)
int64 NumImplicationOnVariableRemoval(BooleanVariable var)
SatClause * ReasonClause(int trail_index) const
SatClause * AddRemovableClause(const std::vector< Literal > &literals, Trail *trail)
void RegisterPropagator(SatPropagator *propagator)
Watcher(SatClause *c, Literal b, int i=2)
void MinimizeConflictExperimental(const Trail &trail, std::vector< Literal > *c)
int64 num_clauses() const
int64 num_removable_clauses() const
bool protected_during_next_cleanup
bool AddBinaryClauseDuringSearch(Literal a, Literal b)
bool IsRemoved(Literal l) const
SatClause * NextClauseToMinimize()
bool TransformIntoMaxCliques(std::vector< std::vector< Literal >> *at_most_ones, int64 max_num_explored_nodes=1e8)
void SetDratProofHandler(DratProofHandler *drat_proof_handler)
void MinimizeConflictFirst(const Trail &trail, std::vector< Literal > *c, SparseBitset< BooleanVariable > *marked)
const VariablesAssignment & Assignment() const
int64 num_literals_removed() const
#define CHECK_EQ(val1, val2)
int64 num_redundant_literals() const
SatClause * InprocessingAddClause(absl::Span< const Literal > new_clause)
ABSL_MUST_USE_RESULT bool AddAtMostOne(absl::Span< const Literal > at_most_one)
LiteralIndex Index() const
bool AddClause(absl::Span< const Literal > literals, Trail *trail)
const Literal *const end() const
Class that owns everything related to a particular optimization model.
void SetDratProofHandler(DratProofHandler *drat_proof_handler)
void RemoveFixedVariables()
Literal SecondLiteral() const
void LazyDetach(SatClause *clause)
void Attach(SatClause *clause, Trail *trail)
LiteralWatchers(Model *model)
int DirectImplicationsEstimatedSize(Literal literal) const
void MinimizeConflictWithReachability(std::vector< Literal > *c)
static SatClause * Create(absl::Span< const Literal > literals)
int64 num_inspected_clauses() const
void CleanupAllRemovedVariables()
int64 num_minimization() const
void AddBinaryClause(Literal a, Literal b)
int64 num_watched_clauses() const
ABSL_MUST_USE_RESULT bool InprocessingRewriteClause(SatClause *clause, absl::Span< const Literal > new_clause)
Literal PropagatedLiteral() const
Literal FirstLiteral() const
Literal RepresentativeOf(Literal l) const
absl::flat_hash_map< SatClause *, ClauseInfo > * mutable_clauses_info()
const Literal *const begin() const
void ChangeReason(int trail_index, int propagator_id)
void Detach(SatClause *clause)
#define DISALLOW_COPY_AND_ASSIGN(TypeName)
std::string StatString() const
bool IsSatisfied(const VariablesAssignment &assignment) const
const std::vector< Watcher > & WatcherListOnFalse(Literal false_literal) const
void AddImplication(Literal a, Literal b)
int64 num_inspected_clause_literals() const
void Resize(int num_variables)
bool operator!=(BinaryClause o) const
int64 num_implications() const
std::mt19937 random_engine_t
bool operator==(BinaryClause o) const
const absl::InlinedVector< Literal, 6 > & Implications(Literal l) const
BinaryClause(Literal _a, Literal _b)
~LiteralWatchers() override
bool ContainsKey(const Collection &collection, const Key &key)