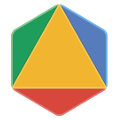 |
OR-Tools
8.1
|
Go to the documentation of this file.
14 #ifndef OR_TOOLS_GLOP_UPDATE_ROW_H_
15 #define OR_TOOLS_GLOP_UPDATE_ROW_H_
67 return coefficient_[
col];
81 std::string
StatString()
const {
return stats_.StatString(); }
89 const std::string& algorithm);
103 void ComputeUnitRowLeftInverse(RowIndex leaving_row);
107 void ComputeUpdatesRowWise();
108 void ComputeUpdatesRowWiseHypersparse();
109 void ComputeUpdatesColumnWise();
123 std::vector<ColIndex> unit_row_left_inverse_filtered_non_zeros_;
132 bool compute_update_row_;
133 RowIndex update_row_computed_for_;
139 unit_row_left_inverse_density(
"unit_row_left_inverse_density", this),
140 unit_row_left_inverse_accuracy(
"unit_row_left_inverse_accuracy",
142 update_row_density(
"update_row_density", this) {}
150 int64 num_operations_;
153 GlopParameters parameters_;
162 #endif // OR_TOOLS_GLOP_UPDATE_ROW_H_
std::string StatString() const
void IgnoreUpdatePosition(ColIndex col)
const Fractional GetCoefficient(ColIndex col) const
The vehicle routing library lets one model and solve generic vehicle routing problems ranging from th...
double DeterministicTime() const
static double DeterministicTimeForFpOperations(int64 n)
void RecomputeFullUpdateRow(RowIndex leaving_row)
void ComputeUpdateRow(RowIndex leaving_row)
std::vector< ColIndex > ColIndexVector
void SetParameters(const GlopParameters ¶meters)
UpdateRow(const CompactSparseMatrix &matrix, const CompactSparseMatrix &transposed_matrix, const VariablesInfo &variables_info, const RowToColMapping &basis, const BasisFactorization &basis_factorization)
const DenseRow & GetCoefficients() const
const ScatteredRow & ComputeAndGetUnitRowLeftInverse(RowIndex leaving_row)
const ScatteredRow & GetUnitRowLeftInverse() const
const ColIndexVector & GetNonZeroPositions() const
void ComputeUpdateRowForBenchmark(const DenseRow &lhs, const std::string &algorithm)