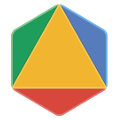 |
OR-Tools
8.1
|
Go to the documentation of this file.
14 #ifndef OR_TOOLS_GLOP_VARIABLES_INFO_H_
15 #define OR_TOOLS_GLOP_VARIABLES_INFO_H_
77 return upper_bound_[
col] - lower_bound_[
col];
85 void SetRelevance(ColIndex
col,
bool relevance);
120 EntryIndex num_entries_in_relevant_columns_;
123 bool boxed_variables_are_relevant_;
131 #endif // OR_TOOLS_GLOP_VARIABLES_INFO_H_
const DenseBitRow & GetNotBasicBitRow() const
VariablesInfo(const CompactSparseMatrix &matrix, const DenseRow &lower_bound, const DenseRow &upper_bound)
void UpdateToBasicStatus(ColIndex col)
Fractional GetBoundDifference(ColIndex col) const
void MakeBoxedVariableRelevant(bool value)
The vehicle routing library lets one model and solve generic vehicle routing problems ranging from th...
const DenseRow & GetVariableUpperBounds() const
ColIndex num_cols() const
const VariableStatusRow & GetStatusRow() const
EntryIndex GetNumEntriesInRelevantColumns() const
const DenseRow & GetVariableLowerBounds() const
const DenseBitRow & GetCanIncreaseBitRow() const
void InitializeAndComputeType()
const ColIndex GetNumberOfColumns() const
const DenseBitRow & GetCanDecreaseBitRow() const
const DenseBitRow & GetIsBasicBitRow() const
const DenseBitRow & GetIsRelevantBitRow() const
void UpdateToNonBasicStatus(ColIndex col, VariableStatus status)
const DenseBitRow & GetNonBasicBoxedVariables() const
const VariableTypeRow & GetTypeRow() const
void Update(ColIndex col, VariableStatus status)