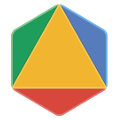 |
OR-Tools
8.1
|
Go to the documentation of this file.
26 #ifndef OR_TOOLS_GSCIP_GSCIP_EXT_H_
27 #define OR_TOOLS_GSCIP_GSCIP_EXT_H_
29 #include "absl/status/status.h"
31 #include "scip/scip.h"
32 #include "scip/scip_prob.h"
33 #include "scip/type_cons.h"
34 #include "scip/type_scip.h"
35 #include "scip/type_var.h"
41 absl::Status
GScipCreateAbs(GScip* gscip, SCIP_Var* x, SCIP_Var* abs_x,
42 const std::string&
name =
"");
47 absl::flat_hash_map<SCIP_VAR*, double>
terms;
70 const std::vector<GScipLinearExpr>& terms,
71 const std::string&
name =
"");
76 const std::vector<GScipLinearExpr>& terms,
77 const std::string&
name =
"");
90 const std::string&
name =
"",
100 GScip* gscip, std::vector<SCIP_Var*> quadratic_variables1,
101 std::vector<SCIP_Var*> quadratic_variables2,
102 std::vector<double> quadratic_coefficients,
const std::string&
name =
"");
106 #endif // OR_TOOLS_GSCIP_GSCIP_EXT_H_
SCIP_VAR * indicator_variable
The vehicle routing library lets one model and solve generic vehicle routing problems ranging from th...
GScipLinearExpr GScipDifference(GScipLinearExpr left, const GScipLinearExpr &right)
absl::Status GScipCreateIndicatorRange(GScip *gscip, const GScipIndicatorRangeConstraint &indicator_range, const std::string &name, const GScipConstraintOptions &options)
absl::Status GScipAddQuadraticObjectiveTerm(GScip *gscip, std::vector< SCIP_Var * > quadratic_variables1, std::vector< SCIP_Var * > quadratic_variables2, std::vector< double > quadratic_coefficients, const std::string &name)
GScipLinearExpr()=default
absl::Status GScipCreateAbs(GScip *gscip, SCIP_Var *x, SCIP_Var *abs_x, const std::string &name)
GScipLinearRange GScipLe(const GScipLinearExpr left, const GScipLinearExpr &right)
absl::Status GScipCreateMaximum(GScip *gscip, const GScipLinearExpr &resultant, const std::vector< GScipLinearExpr > &terms, const std::string &name)
absl::Status GScipCreateMinimum(GScip *gscip, const GScipLinearExpr &resultant, const std::vector< GScipLinearExpr > &terms, const std::string &name)
absl::flat_hash_map< SCIP_VAR *, double > terms
GScipLinearExpr GScipNegate(GScipLinearExpr expr)