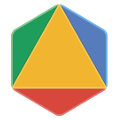 |
OR-Tools
8.1
|
Go to the documentation of this file.
14 #ifndef OR_TOOLS_SAT_RINS_H_
15 #define OR_TOOLS_SAT_RINS_H_
19 #include "absl/container/flat_hash_map.h"
20 #include "absl/synchronization/mutex.h"
21 #include "absl/types/optional.h"
44 std::vector<LPVariable>
vars;
58 std::vector<std::pair< int, std::pair<int64, int64>>>
88 #endif // OR_TOOLS_SAT_RINS_H_
const IntegerVariable kNoIntegerVariable(-1)
LinearProgrammingConstraint * lp
std::vector< LPVariable > vars
The vehicle routing library lets one model and solve generic vehicle routing problems ranging from th...
SharedRelaxationSolutionRepository * relaxation_solutions
RINSNeighborhood GetRINSNeighborhood(const SharedResponseManager *response_manager, const SharedRelaxationSolutionRepository *relaxation_solutions, const SharedLPSolutionRepository *lp_solutions, SharedIncompleteSolutionManager *incomplete_solutions, random_engine_t *random)
std::vector< std::pair< int, std::pair< int64, int64 > > > reduced_domain_vars
SharedLPSolutionRepository * lp_solutions
Class that owns everything related to a particular optimization model.
bool operator==(const LPVariable other) const
std::vector< std::pair< int, int64 > > fixed_vars
IntegerVariable positive_var
SharedIncompleteSolutionManager * incomplete_solutions
std::mt19937 random_engine_t
void RecordLPRelaxationValues(Model *model)