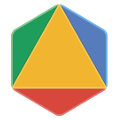 |
OR-Tools
8.1
|
Go to the documentation of this file.
60 #ifndef OR_TOOLS_LP_DATA_MATRIX_SCALER_H_
61 #define OR_TOOLS_LP_DATA_MATRIX_SCALER_H_
111 void Scale(GlopParameters::ScalingAlgorithm method);
157 RowIndex ScaleMatrixRows(
const DenseColumn& factors);
165 ColIndex GetRowScaleIndex(RowIndex row_num);
168 ColIndex GetColumnScaleIndex(ColIndex col_num);
173 std::string DebugInformationString()
const;
194 #endif // OR_TOOLS_LP_DATA_MATRIX_SCALER_H_
const DenseRow & col_scale() const
Fractional ColUnscalingFactor(ColIndex col) const
void ScaleColumnVector(bool up, DenseColumn *column_vector) const
ColIndex ScaleColumnsGeometrically()
void ScaleRowVector(bool up, DenseRow *row_vector) const
The vehicle routing library lets one model and solve generic vehicle routing problems ranging from th...
ColIndex EquilibrateColumns()
Fractional RowUnscalingFactor(RowIndex row) const
void Scale(GlopParameters::ScalingAlgorithm method)
Fractional RowScalingFactor(RowIndex row) const
void Init(SparseMatrix *matrix)
Fractional ColScalingFactor(ColIndex col) const
RowIndex ScaleRowsGeometrically()
RowIndex EquilibrateRows()
Fractional VarianceOfAbsoluteValueOfNonZeros() const