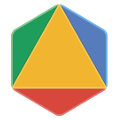 |
OR-Tools
8.1
|
Go to the documentation of this file.
14 #ifndef OR_TOOLS_BOP_INTEGRAL_SOLVER_H_
15 #define OR_TOOLS_BOP_INTEGRAL_SOLVER_H_
67 BopParameters parameters_;
76 #endif // OR_TOOLS_BOP_INTEGRAL_SOLVER_H_
void SetParameters(const BopParameters ¶meters)
glop::Fractional objective_value() const
The vehicle routing library lets one model and solve generic vehicle routing problems ranging from th...
A simple class to enforce both an elapsed time limit and a deterministic time limit in the same threa...
const glop::DenseRow & variable_values() const
SharedTimeLimit * time_limit
ABSL_MUST_USE_RESULT BopSolveStatus SolveWithTimeLimit(const glop::LinearProgram &linear_problem, TimeLimit *time_limit)
BopParameters parameters() const
ABSL_MUST_USE_RESULT BopSolveStatus Solve(const glop::LinearProgram &linear_problem, const glop::DenseRow &user_provided_initial_solution)
ABSL_MUST_USE_RESULT BopSolveStatus SolveWithTimeLimit(const glop::LinearProgram &linear_problem, const glop::DenseRow &user_provided_initial_solution, TimeLimit *time_limit)
ABSL_MUST_USE_RESULT BopSolveStatus Solve(const glop::LinearProgram &linear_problem)
glop::Fractional best_bound() const