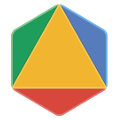 |
OR-Tools
8.1
|
Go to the documentation of this file.
14 #ifndef OR_TOOLS_BASE_HASH_H_
15 #define OR_TOOLS_BASE_HASH_H_
112 template <
class First,
class Second>
113 struct hash<std::pair<First, Second>> {
115 size_t h1 = hash<First>()(p.first);
116 size_t h2 = hash<Second>()(p.second);
118 return (
sizeof(h1) <=
sizeof(
uint32))
125 template <
class T, std::
size_t N>
126 struct hash<std::array<T, N>> {
131 const T& elem = t[
index];
132 const uint64 new_hash = hash<T>()(elem);
138 bool operator()(
const std::array<T, N>&
a,
const std::array<T, N>&
b)
const {
141 static const size_t bucket_size = 4;
142 static const size_t min_buckets = 8;
163 #endif // OR_TOOLS_BASE_HASH_H_
size_t operator()(const std::array< T, N > &t) const
The vehicle routing library lets one model and solve generic vehicle routing problems ranging from th...
static void mix(uint32 &a, uint32 &b, uint32 &c)
bool operator()(const std::array< T, N > &a, const std::array< T, N > &b) const
size_t operator()(const std::pair< First, Second > &p) const
uint64 Hash64NumWithSeed(uint64 num, uint64 c)
uint32 Hash32NumWithSeed(uint32 num, uint32 c)
uint64 Hash(uint64 num, uint64 c)