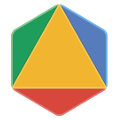 |
OR-Tools
8.1
|
Go to the documentation of this file.
14 #ifndef OR_TOOLS_BOP_BOP_LNS_H_
15 #define OR_TOOLS_BOP_BOP_LNS_H_
50 bool ShouldBeRun(
const ProblemState& problem_state)
const final;
56 const ProblemState& problem_state,
int num_relaxed_vars);
58 int64 state_update_stamp_;
59 std::unique_ptr<sat::SatSolver> sat_solver_;
112 bool ShouldBeRun(
const ProblemState& problem_state)
const final;
117 const bool use_lp_to_guide_sat_;
118 std::unique_ptr<NeighborhoodGenerator> neighborhood_generator_;
132 : objective_terms_(*objective_terms), random_(random) {}
136 void GenerateNeighborhood(
const ProblemState& problem_state,
150 : objective_terms_(*objective_terms), random_(random) {}
154 void GenerateNeighborhood(
const ProblemState& problem_state,
171 void GenerateNeighborhood(
const ProblemState& problem_state,
183 #endif // OR_TOOLS_BOP_BOP_LNS_H_
~ObjectiveBasedNeighborhood() final
RelationGraphBasedNeighborhood(const sat::LinearBooleanProblem &problem, MTRandom *random)
~BopCompleteLNSOptimizer() final
~BopAdaptiveLNSOptimizer() final
const std::string & name() const
~ConstraintBasedNeighborhood() final
The vehicle routing library lets one model and solve generic vehicle routing problems ranging from th...
A simple class to enforce both an elapsed time limit and a deterministic time limit in the same threa...
ConstraintBasedNeighborhood(const BopConstraintTerms *objective_terms, MTRandom *random)
SharedTimeLimit * time_limit
virtual ~NeighborhoodGenerator()
~RelationGraphBasedNeighborhood() final
BopAdaptiveLNSOptimizer(const std::string &name, bool use_lp_to_guide_sat, NeighborhoodGenerator *neighborhood_generator, sat::SatSolver *sat_propagator)
ObjectiveBasedNeighborhood(const BopConstraintTerms *objective_terms, MTRandom *random)
BopCompleteLNSOptimizer(const std::string &name, const BopConstraintTerms &objective_terms)
virtual void GenerateNeighborhood(const ProblemState &problem_state, double difficulty, sat::SatSolver *sat_propagator)=0