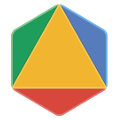 |
OR-Tools
8.1
|
Go to the documentation of this file.
14 #ifndef OR_TOOLS_BASE_BITMAP_H_
15 #define OR_TOOLS_BASE_BITMAP_H_
45 map_(new
uint64[array_size_]) {
59 assert(max_size_ == 0 ||
index < max_size_);
63 assert(max_size_ == 0 ||
index < max_size_);
73 memset(map_, (
value ? 0xFF : 0x00), array_size_ *
sizeof(*map_));
87 #endif // OR_TOOLS_BASE_BITMAP_H_
uint64 BitLength64(uint64 size)
The vehicle routing library lets one model and solve generic vehicle routing problems ranging from th...
uint64 BitPos64(uint64 pos)
void SetBit64(uint64 *const bitset, uint64 pos)
void Set(uint32 index, bool value)
void Resize(uint32 size, bool fill=false)
uint64 BitLength64(uint64 size)
bool IsBitSet64(const uint64 *const bitset, uint64 pos)
void ClearBit64(uint64 *const bitset, uint64 pos)
uint64 BitOffset64(uint64 pos)
bool Get(uint32 index) const
Bitmap(uint32 size, bool fill=false)