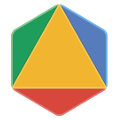 |
OR-Tools
8.1
|
Go to the documentation of this file.
14 #ifndef OR_TOOLS_BASE_FILE_H_
15 #define OR_TOOLS_BASE_FILE_H_
21 #include "absl/status/status.h"
22 #include "absl/strings/string_view.h"
23 #include "google/protobuf/descriptor.h"
24 #include "google/protobuf/io/tokenizer.h"
25 #include "google/protobuf/message.h"
26 #include "google/protobuf/text_format.h"
36 static File*
Open(
const char*
const name,
const char*
const flag);
38 #ifndef SWIG // no overloading
40 const char*
const mode) {
49 #ifndef SWIG // no overloading
51 const char*
const flag) {
57 size_t Read(
void*
const buff,
size_t size);
61 void ReadOrDie(
void*
const buff,
size_t size);
72 size_t Write(
const void*
const buff,
size_t size);
76 void WriteOrDie(
const void*
const buff,
size_t size);
86 absl::Status
Close(
int flags);
112 File(FILE*
const descriptor,
const absl::string_view&
name);
115 const absl::string_view name_;
122 absl::Status
Open(
const absl::string_view& filename,
123 const absl::string_view& mode,
File** f,
int flags);
125 const absl::string_view& mode,
int flags);
126 absl::Status
GetTextProto(
const absl::string_view& filename,
127 google::protobuf::Message*
proto,
int flags);
128 absl::Status
SetTextProto(
const absl::string_view& filename,
129 const google::protobuf::Message&
proto,
int flags);
131 const google::protobuf::Message&
proto,
int flags);
132 absl::Status
SetContents(
const absl::string_view& filename,
133 const absl::string_view& contents,
int flags);
134 absl::Status
GetContents(
const absl::string_view& filename, std::string* output,
139 bool ReadFileToString(
const absl::string_view& file_name, std::string* output);
141 const absl::string_view& file_name);
143 google::protobuf::Message*
proto);
145 google::protobuf::Message*
proto);
147 const absl::string_view& file_name);
149 const absl::string_view& file_name);
151 const absl::string_view& file_name);
153 const absl::string_view& file_name);
155 absl::Status
Delete(
const absl::string_view& path,
int flags);
156 absl::Status
Exists(
const absl::string_view& path,
int flags);
160 #endif // OR_TOOLS_BASE_FILE_H_
absl::Status SetContents(const absl::string_view &filename, const absl::string_view &contents, int flags)
absl::Status GetContents(const absl::string_view &filename, std::string *output, int flags)
absl::Status WriteString(File *file, const absl::string_view &contents, int flags)
absl::Status Exists(const absl::string_view &path, int flags)
absl::Status SetTextProto(const absl::string_view &filename, const google::protobuf::Message &proto, int flags)
absl::string_view filename() const
bool ReadFileToProto(const absl::string_view &file_name, google::protobuf::Message *proto)
static File * Open(const absl::string_view &name, const char *const mode)
size_t WriteString(const std::string &line)
absl::Status GetTextProto(const absl::string_view &filename, google::protobuf::Message *proto, int flags)
static bool Delete(const char *const name)
bool ReadFileToString(const absl::string_view &file_name, std::string *output)
char * ReadLine(char *const output, uint64 max_length)
bool WriteStringToFile(const std::string &data, const absl::string_view &file_name)
bool WriteLine(const std::string &line)
void ReadOrDie(void *const buff, size_t size)
absl::Status SetBinaryProto(const absl::string_view &filename, const google::protobuf::Message &proto, int flags)
size_t Read(void *const buff, size_t size)
void WriteProtoToFileOrDie(const google::protobuf::Message &proto, const absl::string_view &file_name)
void ReadFileToProtoOrDie(const absl::string_view &file_name, google::protobuf::Message *proto)
bool WriteProtoToFile(const google::protobuf::Message &proto, const absl::string_view &file_name)
static bool Delete(const absl::string_view &name)
size_t Write(const void *const buff, size_t size)
absl::Status Delete(const absl::string_view &path, int flags)
void WriteOrDie(const void *const buff, size_t size)
static File * OpenOrDie(const char *const name, const char *const flag)
absl::Status Open(const absl::string_view &filename, const absl::string_view &mode, File **f, int flags)
int64 ReadToString(std::string *const line, uint64 max_length)
bool WriteProtoToASCIIFile(const google::protobuf::Message &proto, const absl::string_view &file_name)
void WriteProtoToASCIIFileOrDie(const google::protobuf::Message &proto, const absl::string_view &file_name)
File * OpenOrDie(const absl::string_view &filename, const absl::string_view &mode, int flags)
static File * OpenOrDie(const absl::string_view &name, const char *const flag)
static bool Exists(const char *const name)