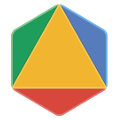 |
OR-Tools
8.1
|
Go to the documentation of this file.
14 #ifndef OR_TOOLS_GSCIP_GSCIP_PARAMETERS_H_
15 #define OR_TOOLS_GSCIP_GSCIP_PARAMETERS_H_
17 #include "absl/time/time.h"
56 #endif // OR_TOOLS_GSCIP_GSCIP_PARAMETERS_H_
bool GScipRandomSeedSet(const GScipParameters ¶meters)
int GScipLogLevel(const GScipParameters ¶meters)
The vehicle routing library lets one model and solve generic vehicle routing problems ranging from th...
bool GScipTimeLimitSet(const GScipParameters ¶meters)
absl::Duration GScipTimeLimit(const GScipParameters ¶meters)
int GScipRandomSeed(const GScipParameters ¶meters)
SharedTimeLimit * time_limit
bool GScipOutputEnabled(const GScipParameters ¶meters)
void GScipSetLogLevel(GScipParameters *parameters, int log_level)
bool GScipLogLevelSet(const GScipParameters ¶meters)
bool GScipMaxNumThreadsSet(const GScipParameters ¶meters)
void GScipSetOutputEnabled(GScipParameters *parameters, bool output_enabled)
int GScipMaxNumThreads(const GScipParameters ¶meters)
void GScipSetRandomSeed(GScipParameters *parameters, int random_seed)
void GScipSetTimeLimit(absl::Duration time_limit, GScipParameters *parameters)
bool GScipOutputEnabledSet(const GScipParameters ¶meters)
void GScipSetMaxNumThreads(int num_threads, GScipParameters *parameters)